When should I use “this” keyword?
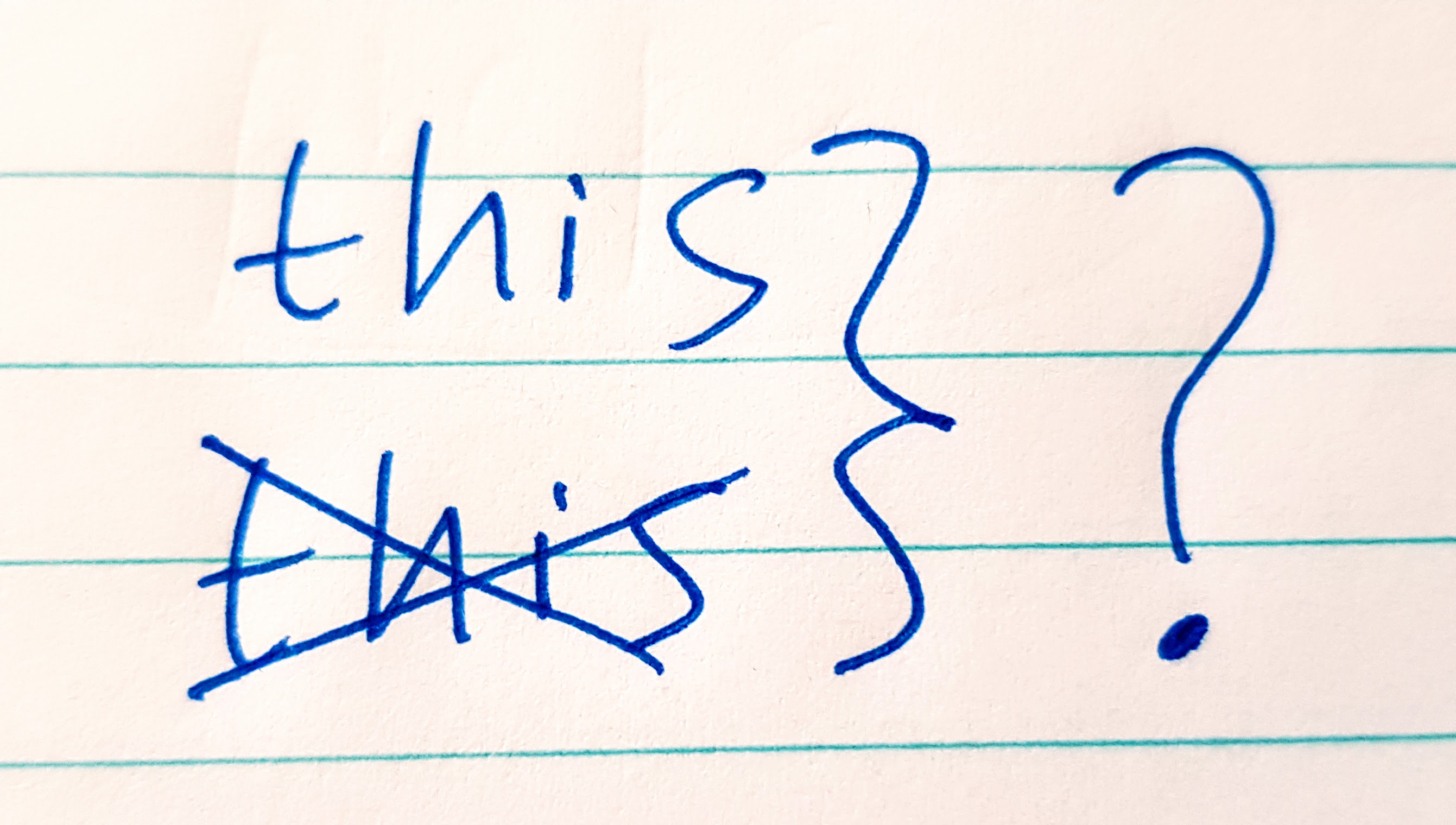
When you browse through Javascript code, you often see function calls prepended with this.
keyword, like this.functionName()
. However, sometimes this
is missing and it’s just functionName()
. What is the difference and when you should use one over the other?
That depends on whether you React component is functional or class-based.
If your component is a class-based component and functionName
is a method of that class, then you must address to it as this.functionName
. For example:
class App extends React.Component {
// myFunc is a method of App class
myfunc() {
...
}
render() {
myfunc(); // Error! "myfunc is not defined"
this.myfunc(); // #ThumbsUp
...
}
}
On the other hand, if your component is functional, then functions you declare inside it do not require this
keyword to be addressed to, no matter if they are defined with function
keyword or as arrow functions.
const App = () => {
function myfunc1(){
...
}
const myfunc2 = () => {
...
}
myfunc1(); // This works
myfunc2(); // This works too
this.myfunc1(); // Error: Cannot read property 'myfunc1' of undefined
...
};